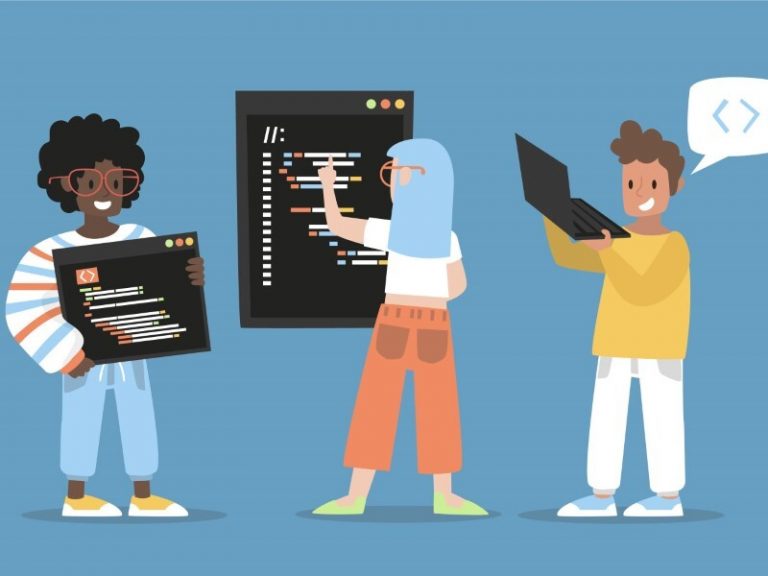
How can we replace all occurrences of a string in JavaScript?
Replacing all occurrences of a particular string in JavaScript is a common problem when working with text data. We can do By applying a variety of techniques and methods.
By applying String replace () method
The most straightforward way to replace all occurrences of a string in JavaScript is by By applying the replace() method. This method is available on string objects and takes two arguments: the substring to be replaced and the replacement string. Here’s an example:
const originalString = 'This is a sample string with some sample text.'; const searchString = 'sample'; const replacementString = 'example'; const modifiedString = originalString.replace(new RegExp(searchString, 'g'), replacementString); console.log(modifiedString);
In this example, we use the replace() method with a regular expression that has the ‘g’ flag, which stands for “global,” ensuring that all occurrences of the search string are replaced. Without the ‘g’ flag, only the first occurrence is replaced.
By applying Regular Expressions
Regular expressions offer more flexibility when replacing all occurrences of a string. You can use them in combination with the replace() method or directly through regular expression methods.
const originalString = 'This is a sample string with some sample text.'; const searchString = 'sample'; const replacementString = 'example'; const regex = new RegExp(searchString, 'g'); const modifiedString = originalString.replace(regex, replacementString); console.log(modifiedString);
Another approach is By applying the String.prototype.split() method followed by Array.prototype.join():
const originalString = 'This is a sample string with some sample text.'; const searchString = 'sample'; const replacementString = 'example'; const parts = originalString.split(searchString); const modifiedString = parts.join(replacementString); console.log(modifiedString);
By applying a Loop
For more control and custom logic during replacements, you can use a loop. This approach is especially useful when you need to replace substrings conditionally or perform more complex replacements:
function replaceAllOccurrences(originalString, searchString, replacementString) { let result = originalString; while (result.includes(searchString)) { result = result.replace(searchString, replacementString); } return result; } const originalString = 'This is a sample string with some sample text.'; const searchString = 'sample'; const replacementString = 'example'; const modifiedString = replaceAllOccurrences(originalString, searchString, replacementString); console.log(modifiedString);
This custom function repeatedly uses the replace() method until no more occurrences of the search string are found.
By applying a Library or Framework
In more complex scenarios, especially when dealing with large datasets or advanced text processing, you might consider By applying third-party libraries or frameworks like lodash, underscore.js, or even regular expression libraries to streamline the process.
Performance Considerations
When replacing all occurrences of a string, it’s essential to consider performance, especially when dealing with large strings or a high number of replacements. By applying regular expressions with the ‘g’ flag is often the most efficient method. The loop-based approach can be slow for extensive replacements.
Case-Insensitive Replacement
If you need to perform a case-insensitive replacement, you can modify the regular expression as follows:
const originalString = 'This is a sample string with some Sample text.'; const searchString = 'sample'; const replacementString = 'example'; const regex = new RegExp(searchString, 'gi'); // 'i' flag stands for case-insensitive const modifiedString = originalString.replace(regex, replacementString); console.log(modifiedString);
In this example, the ‘i’ flag is added to the regular expression to make the replacement case insensitive.
See this also –
- How to include a JavaScript file in another JavaScript file?
- Loop (for еach) ovеr an array in JavaScript
- How do I remove a property from a JavaScript object?
- How can we replace all occurrences of a string in JavaScript?
- Which equals operator (== vs ===) should be used and where in JavaScript comparison ?